Creating additional indows
最後のチュートリアル「Creating additional windows」を実行してみます。
Creating a new window
ボタンをクリックすると子ウインドウが表示されるサンプルコードを実行してみます。
MainWindowクラス(親クラスはQMainWindow)と子ウインドウになるAnotherWindowクラス(親クラスはQWidget)を作成し、
MainWindowクラス内でAnotherWindowをインスタンスし、実行しています。
- import sys
- from PySide6.QtWidgets import (
- QApplication,
- QLabel,
- QMainWindow,
- QPushButton,
- QVBoxLayout,
- QWidget,
- )
- class AnotherWindow(QWidget):
- """
- This "window" is a QWidget. If it has no parent, it
- will appear as a free-floating window as we want.
- """
- def __init__(self):
- super().__init__()
- layout = QVBoxLayout()
- self.label = QLabel("Another Window")
- layout.addWidget(self.label)
- self.setLayout(layout)
- class MainWindow(QMainWindow):
- def __init__(self):
- super().__init__()
- self.button = QPushButton("Push for Window")
- self.button.clicked.connect(self.show_new_window)
- self.setCentralWidget(self.button)
- def show_new_window(self, checked):
- self.w = AnotherWindow()
- self.w.show()
- app = QApplication(sys.argv)
- window = MainWindow()
- window.show()
- app.exec()
実行結果
実行するとまずメインウインドウが生成され、「Push for Window」をクリックすると、子ウインドウとして「Another Window」が表示されました。
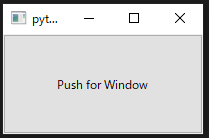
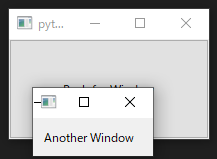
AnotherWindowクラスの__init__にラベルで0から100までの値を表示するように変更。
def __init__(self):
super().__init__()
layout = QVBoxLayout()
self.label = QLabel("Another Window % d" % randint(0, 100))
layout.addWidget(self.label)
self.setLayout(layout)
MainWindowクラスの__init__にself.w=Noneにし、show_new_windowでNoneとNone出ない場合の記述を追加したサンプルコードを実行してみます。
def show_new_window(self, checked):
if self.w is None:
self.w = AnotherWindow()
self.w.show()
else:
self.w.close() # Close window.
self.w = None # Discard reference.
実行結果
「Push for Window」をクリックすると、子ウィンドウが表示されランダム値が85表示されました。もう一度「Push for Window」をクリックすると、子ウインドウは閉じ、さらにもう一回クリックすると今度は33という値で子ウインドウが開きました。
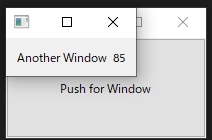
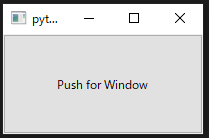

President Windows
次は閉じない子ウインドウのサンプルコードです。
show_new_windowメソッドを時こうした際にself.w.show()のみにすると、クリックしても子ウインドウは閉じませんでした。
def show_new_window(self, checked):
self.w.show()
Showing & hiding persistent windows
次はPresident Windowsのshow&Hide のサンプルコードです。
- import sys
- from random import randint
- from PySide6.QtWidgets import (
- QApplication,
- QLabel,
- QMainWindow,
- QPushButton,
- QVBoxLayout,
- QWidget,
- )
- class AnotherWindow(QWidget):
- """
- This "window" is a QWidget. If it has no parent, it
- will appear as a free-floating window as we want.
- """
- def __init__(self):
- super().__init__()
- layout = QVBoxLayout()
- self.label = QLabel("Another Window % d" % randint(0, 100))
- layout.addWidget(self.label)
- self.setLayout(layout)
- class MainWindow(QMainWindow):
- def __init__(self):
- super().__init__()
- self.w = AnotherWindow()
- self.button = QPushButton("Push for Window")
- self.button.clicked.connect(self.toggle_window)
- self.setCentralWidget(self.button)
- def toggle_window(self, checked):
- if self.w.isVisible():
- self.w.hide()
- else:
- self.w.show()
- app = QApplication(sys.argv)
- window = MainWindow()
- window.show()
- app.exec()
実行結果
「Push for Window」をクリックすると子ウインドウが表示されますが、
もう一度「Push for Window」をクリックするとディスプレイ上から見えなくなります(隠れます。)
さらにもう一回クリックすると子ウインドウが表示されました。

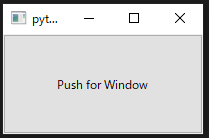
Multiple windows
最後はMulitple windowsです。
AnotherWindowクラスをwindow1とwindow2という2つにインスタンスし、それぞれのボタンをクリックすると対応するwindowが表示されるサンプルコードです。
- import sys
- from random import randint
- from PySide6.QtWidgets import (
- QApplication,
- QLabel,
- QMainWindow,
- QPushButton,
- QVBoxLayout,
- QWidget,
- )
- class AnotherWindow(QWidget):
- """
- This "window" is a QWidget. If it has no parent,
- it will appear as a free-floating window.
- """
- def __init__(self):
- super().__init__()
- layout = QVBoxLayout()
- self.label = QLabel("Another Window % d" % randint(0, 100))
- layout.addWidget(self.label)
- self.setLayout(layout)
- class MainWindow(QMainWindow):
- def __init__(self):
- super().__init__()
- self.window1 = AnotherWindow()
- self.window2 = AnotherWindow()
- layout = QVBoxLayout()
- button1 = QPushButton("Push for Window 1")
- button1.clicked.connect(self.toggle_window1)
- layout.addWidget(button1)
- button2 = QPushButton("Push for Window 2")
- button2.clicked.connect(self.toggle_window2)
- layout.addWidget(button2)
- w = QWidget()
- w.setLayout(layout)
- self.setCentralWidget(w)
- def toggle_window1(self, checked):
- if self.window1.isVisible():
- self.window1.hide()
- else:
- self.window1.show()
- def toggle_window2(self, checked):
- if self.window2.isVisible():
- self.window2.hide()
- else:
- self.window2.show()
- app = QApplication(sys.argv)
- window = MainWindow()
- window.show()
- app.exec()
実行結果
それぞれのボタンをクリックすると子ウインドウがが起動することが確認できました。
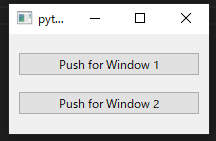
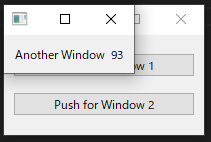
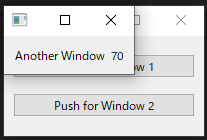
最後のサンプルコードはlambdaを使って引数を動的にわたすというサンプルコードです。結果は同じになります。
layout = QVBoxLayout()
button1 = QPushButton("Push for Window 1")
button1.clicked.connect(
lambda checked: self.toggle_window(self.window1),
)
layout.addWidget(button1)